Essential Concepts and Skills for Backend Developers
Listen to article Podcast:
Becoming a backend developer requires mastering a diverse range of essential skills and concepts. From understanding backend programming languages like Python, Java, and Node.js to managing databases with SQL and NoSQL, the journey to becoming a successful backend developer is filled with key technical knowledge. Whether you're learning how to develop RESTful APIs, optimizing server-side logic, or preparing for a backend developer interview, this comprehensive guide will cover everything you need. You'll explore microservices architecture, security practices, caching strategies, and best practices for creating scalable, efficient applications—all critical components for anyone pursuing a career in backend development.
Backend development forms the backbone of modern web applications. It handles the server-side logic, database management, and API communication that enable the frontend to function effectively. Whether you're building large-scale enterprise solutions or small applications, a strong understanding of backend development is crucial for creating efficient, scalable, and secure systems.
In this guide, we’ll cover the core skills and concepts every backend developer should master, focusing on both theory and practical application.
Core Skills for Backend Developers
1. Programming Languages
Backend development requires proficiency in at least one programming language. The choice of language often depends on the project’s requirements, team preferences, and ecosystem support. Here are some key languages to focus on:
Python: Known for simplicity and readability, Python is great for both web development (with frameworks like Django and Flask) and data-heavy applications.
Java: Widely used in enterprise environments, Java is robust, scalable, and has excellent tooling for building large-scale applications.
Node.js: Built on JavaScript, Node.js is event-driven and perfect for real-time applications like chat apps or games. It allows for full-stack development using a single language—JavaScript.
Ruby: Popularized by the Ruby on Rails framework, Ruby is known for its developer-friendly syntax and rapid development cycles.
Object-Oriented and Functional Programming Paradigms
Backend developers should be familiar with both Object-Oriented Programming (OOP) and Functional Programming paradigms:
OOP: Focuses on organizing code into objects, encapsulating data, and behavior. It promotes reusability and scalability.
Functional Programming: Emphasizes immutability and stateless functions, leading to easier testing and debugging, especially in distributed systems.
2. Database Management
Backend applications often need to interact with databases to store and retrieve data. There are two main types of databases you need to understand:
SQL Databases: Relational databases like MySQL, PostgreSQL, and SQLite use structured query language (SQL) for defining and manipulating data in tabular form. They are best suited for structured data and complex queries.
NoSQL Databases: Examples include MongoDB, Cassandra, and Redis. These databases offer flexible schemas and are great for handling unstructured or semi-structured data at scale.
ORM (Object-Relational Mapping)
Using an ORM like SQLAlchemy (Python) or Sequelize (Node.js) can simplify database interactions. ORMs allow developers to interact with the database using the native language of their application, reducing the need for raw SQL queries.
3. API Development
Most backend systems expose their functionality through APIs (Application Programming Interfaces), allowing frontend applications or other services to interact with them.
RESTful APIs: The most common API design pattern, REST uses HTTP methods like GET, POST, PUT, and DELETE for client-server communication. REST emphasizes statelessness and resource-based URLs.
GraphQL: An alternative to REST, GraphQL allows clients to request only the data they need, making it more efficient in certain scenarios, especially when dealing with complex or nested data structures.
4. Server Management and Deployment
Understanding how to manage and deploy applications on servers is critical for backend developers:
Web Servers: Servers like Apache and Nginx handle incoming web traffic, forwarding requests to the backend application.
Containerization: Tools like Docker allow applications to run in isolated environments, ensuring consistency across different machines.
Orchestration Platforms: Kubernetes helps manage and scale containerized applications by automating deployment, scaling, and maintenance tasks.
5. Security Practices
Security is paramount in backend development. Common security measures include:
Authentication and Authorization: Techniques such as JWT (JSON Web Tokens) and OAuth ensure that only authorized users have access to certain resources.
Input Validation: Ensuring that input from users is correctly sanitized can prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS).
Encryption: Use encryption (e.g., SSL/TLS) to protect sensitive data in transit and at rest.
6. Testing and CI/CD
Testing ensures that the backend works as expected and reduces the chances of bugs in production:
Unit Testing: Tools like Jest (JavaScript) or PyTest (Python) help test individual components of the application in isolation.
Integration Testing: Focuses on testing the interaction between different modules of the application.
CI/CD: Continuous Integration and Continuous Deployment (CI/CD) pipelines automate testing and deployment. Popular CI/CD tools include Jenkins, GitHub Actions, and CircleCI.
7. Error Handling and Logging
Effective error handling and logging are essential for maintaining the health and performance of a backend system:
Error Handling: Catching and handling errors gracefully prevents the application from crashing and provides helpful feedback to users or developers.
Logging: Tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus for logging and monitoring help track application performance, troubleshoot issues, and analyze user behavior.
Key Concepts to Understand
1. Microservices Architecture
Microservices architecture involves designing applications as a collection of small, independent services that communicate over APIs. This approach enhances scalability, fault tolerance, and maintainability, allowing teams to work on different services simultaneously without affecting the entire application.
2. Data Structures and Algorithms
A strong understanding of data structures (e.g., arrays, linked lists, trees) and algorithms is vital for optimizing code performance. Key algorithms include sorting, searching, and graph traversal techniques, all of which are commonly encountered in technical interviews and real-world applications.
3. Asynchronous Programming
Backend applications often need to handle multiple tasks concurrently. Asynchronous programming enables non-blocking I/O operations, improving the efficiency of applications that deal with high traffic or long-running tasks.
Callbacks: A basic form of asynchronous programming.
Promises and async/await: Modern constructs in JavaScript and Python that simplify handling asynchronous code.
4. Caching Strategies
Caching stores copies of data to reduce the load on databases and speed up application response times. Tools like Redis and Memcached are popular for in-memory caching. Effective caching strategies can drastically improve performance in high-traffic systems.
5. Authentication vs. Authorization
Authentication verifies a user's identity (e.g., login systems), while authorization determines what resources a user can access. Both are essential for building secure applications and preventing unauthorized access.
6. Request/Response Lifecycle
Understanding how a request flows from the client to the server and back helps backend developers optimize performance, handle errors properly, and manage state efficiently. This lifecycle includes:
Client request (e.g., browser or mobile app)
Server processing (e.g., routing, middleware)
Database interaction
Server response (e.g., JSON data or HTML content)
Practical Applications
To solidify these concepts, here are some practical projects you can undertake:
Build a RESTful API
: Use Express.js (Node.js) or Flask (Python) to create a simple API that handles CRUD operations.
Full-Stack Project: Combine a frontend framework (e.g.,React) with a Node.js or Django backend. This will give you experience in managing frontend-backend communication.
JWT Authentication
: Implement user authentication and authorization using JWTs in a sample application.
CI/CD Pipeline: Set up a CI/CD pipeline using GitHub Actions or Jenkins to automate your testing and deployment processes.
Conclusion
Backend development requires a strong grasp of various programming languages, frameworks, databases, security practices, and system design principles. By mastering these skills and concepts, you can build robust, scalable, and secure applications that meet modern web standards.
This comprehensive guide should serve as a roadmap for backend developers, offering insight into the essential knowledge and tools required to excel in this domain.
Further Reading:
By continually learning and applying these essential concepts, you’ll be well-equipped to thrive as a backend developer in any environment!
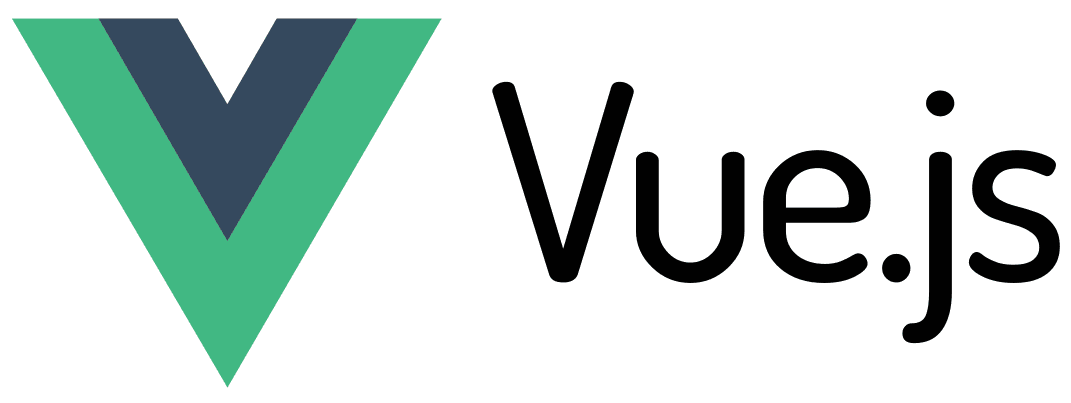
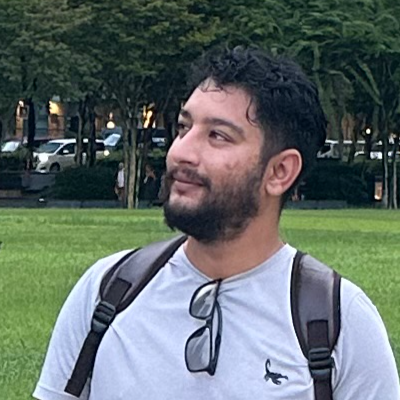
Kiran Chaulagain
kkchaulagain@gmail.com
I am a Full Stack Software Engineer and DevOps expert with over 6 years of experience. Specializing in creating innovative, scalable solutions using technologies like PHP, Node.js, Vue, React, Docker, and Kubernetes, I have a strong foundation in both development and infrastructure with a BSc in Computer Science and Information Technology (CSIT) from Tribhuvan University. I’m passionate about staying ahead of industry trends and delivering projects on time and within budget, all while bridging the gap between development and production. Currently, I’m exploring new opportunities to bring my skills and expertise to exciting new challenges.