How to Easily Dockerize a Python Flask Application: A Step-by-Step Guide
Listen to article podcast:
How to Easily Dockerize a Python Flask Application: A Step-by-Step Guide
Containerization has transformed the way developers build, ship, and run applications. Docker allows you to package your application with all its dependencies, ensuring consistent performance across different environments. In this article, we’ll walk you through the simplest way to Dockerize a Python Flask application, from building a Dockerfile to deploying your app. Along the way, we’ll cover the advantages and potential pitfalls to watch out for when using Docker with Flask.
Why Dockerize Your Flask Application?
Flask is a lightweight and popular web framework for Python, and Docker offers a powerful tool to manage your application’s environment. Dockerizing a Flask application brings several benefits, including:
Consistency: Docker ensures the application behaves the same way in development, staging, and production.
Portability: You can run your Flask app anywhere, as long as Docker is installed.
Scalability: Docker makes it easier to scale your application with container orchestration tools like Kubernetes.
Now, let’s get into how you can quickly Dockerize your Flask app.
Step-by-Step Guide to Dockerizing a Flask App
1. Create a Simple Flask Application
If you don’t already have a Flask app, here’s a basic version to get started. Create a file called app.py with the following content:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask with Docker!"
if __name__ == "__main__":
app.run(host="0.0.0.0", port=5000)
2. Manage Dependencies with requirements.txt
List your application’s dependencies in a requirements.txt file:pip freeze > requirements.txtFor a simple app like this, it might look like this:
Flask==2.0.3
3. Create the Dockerfile
Next, we create a Dockerfile, which contains instructions for building the Docker image. Here’s a basic Dockerfile for Flask:# Use a lightweight Python image
FROM python:3.9-slim
# Set working directory inside the container
WORKDIR /app
# Copy the current directory contents into the container
COPY . /app
# Install the dependencies
RUN pip install -r requirements.txt
# Expose the port Flask will run on
EXPOSE 5000
# Define the command to start the app
CMD ["python", "app.py"]
This Dockerfile starts by pulling the official Python image, installs the necessary packages, and then runs the Flask app.
4. Optimize with a .dockerignore File
To keep the image clean and avoid unnecessary files, create a .dockerignore file:
__pycache__ *.pyc *.pyo .git
This file ensures that temporary files and unnecessary directories are excluded from the Docker image.
5. Build the Docker Image
Once everything is set up, build the Docker image with the following command:
docker build -t flask-app .
This will create a Docker image named flask-app.
6. Run the Flask Application in a Docker Container
After building the image, run your Flask app in a container:docker run -p 5000:5000 flask-appThis maps port 5000 inside the container to port 5000 on your host machine. Open a browser and go to http://localhost:5000 — you should see "Hello, Flask with Docker!"
Taking It Further: Production-Ready Dockerization
For production, you’ll want to optimize your Docker setup. Here are a few best practices:
1. Use Gunicorn for Production Servers
Flask’s built-in server is not suitable for production. Instead, use Gunicorn to handle multiple requests more efficiently. Update the Dockerfile:
CMD ["gunicorn", "-w", "4", "-b", "0.0.0.0:5000", "app:app"]
This tells Docker to use Gunicorn with 4 worker processes.
2. Consider Using Docker Compose
If your Flask app depends on other services, such as a database, Docker Compose makes it easy to manage multi-container applications. Here’s a basic docker-compose.yml file:
version: "3.8"
services:
flask-app:
build: .
ports:
- "5000:5000"
environment:
- FLASK_ENV=production
You can scale your app or add other services like PostgreSQL or Redis easily with Docker Compose.
Pros of Dockerizing a Flask Application
Consistency Across Environments: Docker eliminates issues related to differing environments by packaging the application with all its dependencies.
Isolation: Each Docker container runs in its isolated environment, ensuring no interference with other apps on the host machine.
Portability: Once Dockerized, your Flask app can run on any system that supports Docker — whether it’s a local machine, a server, or a cloud environment.
Scaling Made Easy: With Docker, you can quickly scale the number of containers running your app to handle more traffic.
Streamlined Deployment: Docker simplifies the deployment process by ensuring that your app behaves the same way across all stages — from development to production.
Cons of Dockerizing a Flask Application
Learning Curve: For developers new to Docker, understanding the container ecosystem can take some time.
Performance Overhead: While Docker is more efficient than traditional virtual machines, it still introduces slight overhead compared to running applications natively
Storage and Networking Management: Persistent storage and managing complex networking between containers can require extra configuration and knowledge.
Security Risks: Containers are not as secure as full virtual machines. It’s essential to follow best practices like not running containers as root and keeping images up-to-date.
Resource Consumption: Containers, while lightweight, still require system resources. Running multiple containers on a single machine may lead to performance bottlenecks.
Conclusion: Why Dockerize Your Flask App?
Dockerizing a Flask app streamlines both development and deployment, offering a consistent and portable environment. By following the steps outlined above, you can easily create a Docker image of your Flask application, making it ready for production deployment or scalable across different environments.With its numerous benefits like consistency, portability, and ease of scaling, Docker has become an essential tool for modern application development. While there are some considerations regarding learning curves and resource management, the advantages of Dockerizing your Flask app far outweigh the downsides.Take the plunge into Docker, and watch your Flask applications scale seamlessly across environments!
By following these steps, you’re ready to Dockerize your Flask app and harness the power of containerization. Whether you’re building a small personal project or an enterprise-level web app, Docker provides the tools to simplify your development process and ensure consistent, reliable deployments.
Ready to get started? Dockerize your Flask app today!
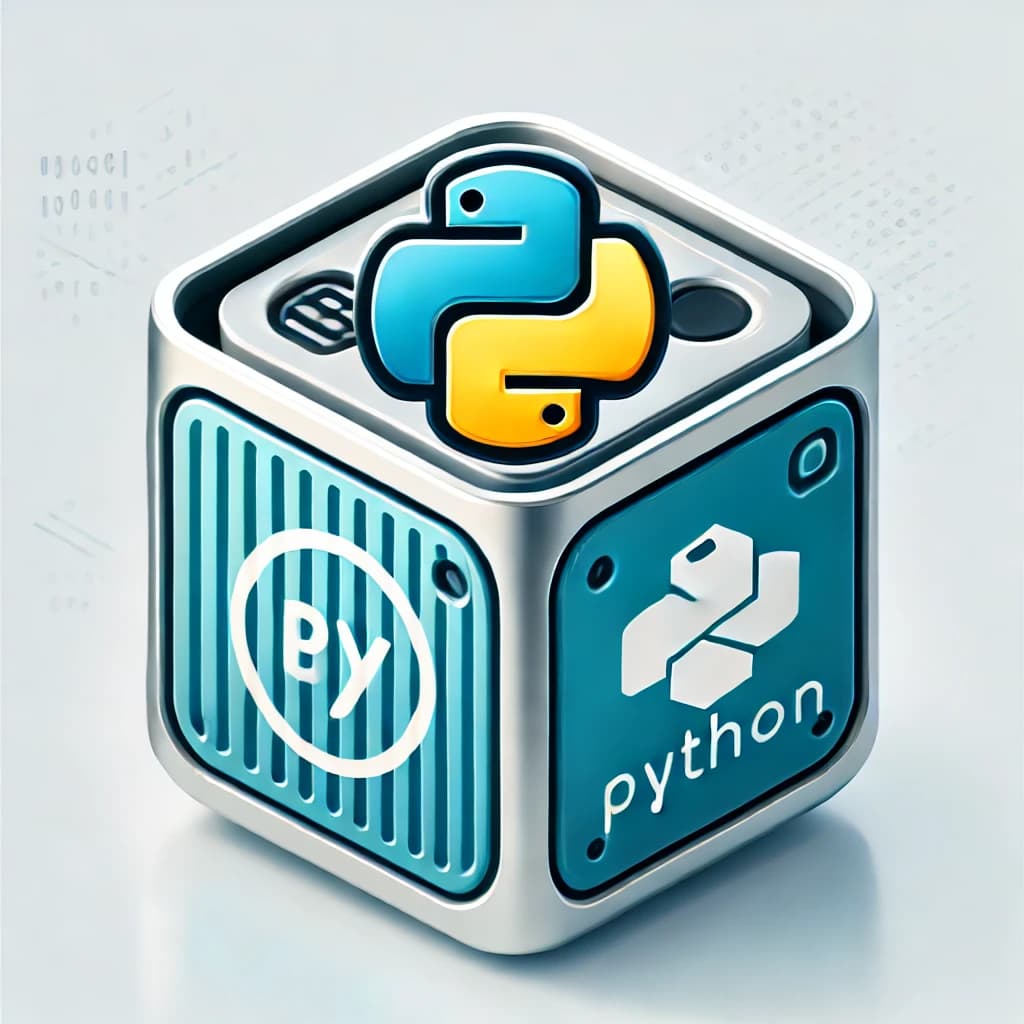
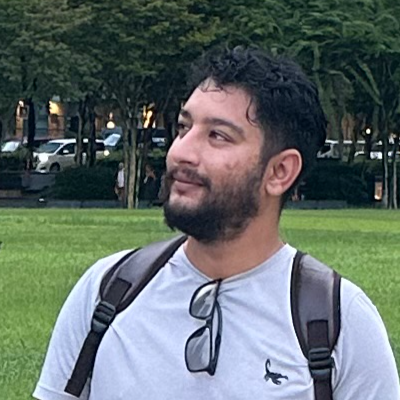
Kiran Chaulagain
kkchaulagain@gmail.com
I am a Full Stack Software Engineer and DevOps expert with over 6 years of experience. Specializing in creating innovative, scalable solutions using technologies like PHP, Node.js, Vue, React, Docker, and Kubernetes, I have a strong foundation in both development and infrastructure with a BSc in Computer Science and Information Technology (CSIT) from Tribhuvan University. I’m passionate about staying ahead of industry trends and delivering projects on time and within budget, all while bridging the gap between development and production. Currently, I’m exploring new opportunities to bring my skills and expertise to exciting new challenges.