Integrating Google Analytics with Next.js: A Step-by-Step Guide
Learn how to integrate Google Analytics with your Next.js application in this step-by-step guide. Improve your website’s performance and user engagement with powerful analytics tools.
In today’s data-driven world, understanding user behavior on your website is crucial for making informed decisions. Google Analytics is a powerful tool that provides valuable insights into your site’s performance and user engagement. If you’re using Next.js for your web development projects, you’ll be pleased to know that integrating Google Analytics is a straightforward process. In this guide, we’ll walk you through the steps to set up Google Analytics in your Next.js application.
Prerequisites
Before we begin, make sure you have:
A Next.js project set up
A Google Analytics 4 (GA4) property created
Your Google Analytics Measurement ID (starts with “G-”)
Step 1: Install the Required Package
First, we need to install the
@next/third-parties
npm install @next/third-parties
Step 2: Create a Shared Layout Component
To ensure Google Analytics is loaded on all pages, we’ll create a shared Layout component. Create a new file
components/Layout.tsx
import { GoogleAnalytics } from '@next/third-parties/google'
import { ReactNode } from 'react'
import { usePageViews } from '@/hooks/usePageViews'interface LayoutProps {
children: ReactNode
}export default function Layout({ children }: LayoutProps) {
usePageViews() // Use the custom hook for page view tracking return (
<>
{children}
{process.env.NEXT_PUBLIC_GA_ID && (
<GoogleAnalytics gaId={process.env.NEXT_PUBLIC_GA_ID} />
)}
</>
)
}
Step 3: Create a Custom Hook for Page View Tracking
Next, let’s create a custom hook to track page views. Add a new file
hooks/usePageViews.ts
import { useEffect } from 'react'
import { useRouter } from 'next/router'
export const usePageViews = () => {
const router = useRouter()
useEffect(() => {
const handleRouteChange = (url: string) => {
if (process.env.NEXT_PUBLIC_GA_ID) {
window.gtag('config', process.env.NEXT_PUBLIC_GA_ID, {
page_path: url,
})
}
}
router.events.on('routeChangeComplete', handleRouteChange)
return () => {
router.events.off('routeChangeComplete', handleRouteChange)
}
}, [router.events])
}
Step 4: Update Your Pages
Now, update your pages to use the shared Layout component. For example, in your
pages/index.tsx
import Layout from "@/components/Layout";
import SeoComponent from "@/components/SeoComponent";
const Home = ({ homepage, isArticle, seo }: any) => {
// Your existing component logic here
return (
<Layout>
{/* Your page content */}
{seo && <SeoComponent data={seo} />}
</Layout>
);
};
export default Home;
Step 5: Set Up Environment Variables
To keep your Google Analytics ID secure and easily configurable, use an environment variable. Create a
.env.local
NEXT_PUBLIC_GA_ID=G-XXXXXXXXXX
Replace
G-XXXXXXXXXX
Step 6: Update next.config.js
Ensure your
next.config.js
module.exports = {
// ... (your existing config)
images: {
domains: ['www.googletagmanager.com'],
},
}
Conclusion
By following these steps, you’ve successfully integrated Google Analytics into your Next.js application. This setup allows you to track page views and user interactions across your site, providing valuable data for improving user experience and achieving your business goals.
Remember to test your implementation thoroughly and check your Google Analytics dashboard to ensure data is being collected correctly. Happy analyzing!
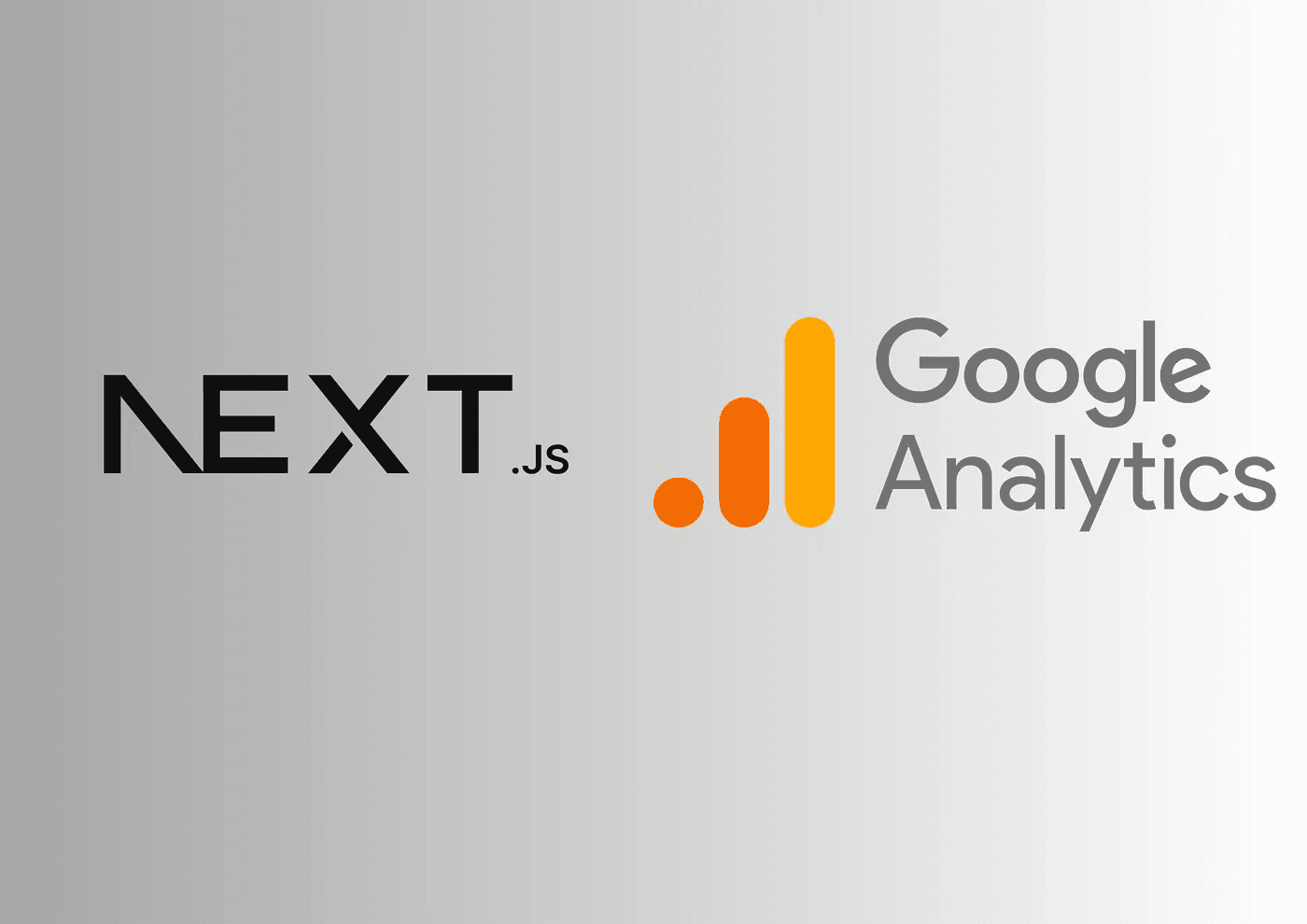
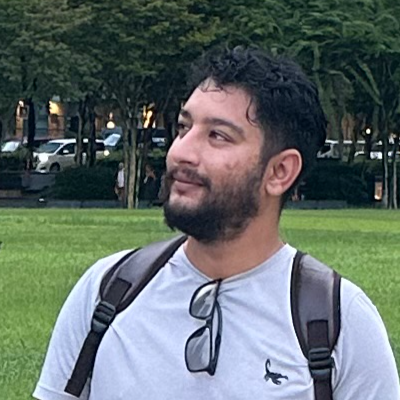
Kiran Chaulagain
kkchaulagain@gmail.com
I am a Full Stack Software Engineer and DevOps expert with over 6 years of experience. Specializing in creating innovative, scalable solutions using technologies like PHP, Node.js, Vue, React, Docker, and Kubernetes, I have a strong foundation in both development and infrastructure with a BSc in Computer Science and Information Technology (CSIT) from Tribhuvan University. I’m passionate about staying ahead of industry trends and delivering projects on time and within budget, all while bridging the gap between development and production. Currently, I’m exploring new opportunities to bring my skills and expertise to exciting new challenges.