Mastering Server-Side Rendering with React and Next.js for Optimized Web Applications
Listen to article Podcast
Boost Your React App Performance and SEO: A Step-by-Step Guide to Mastering Server-Side Rendering with Next.js
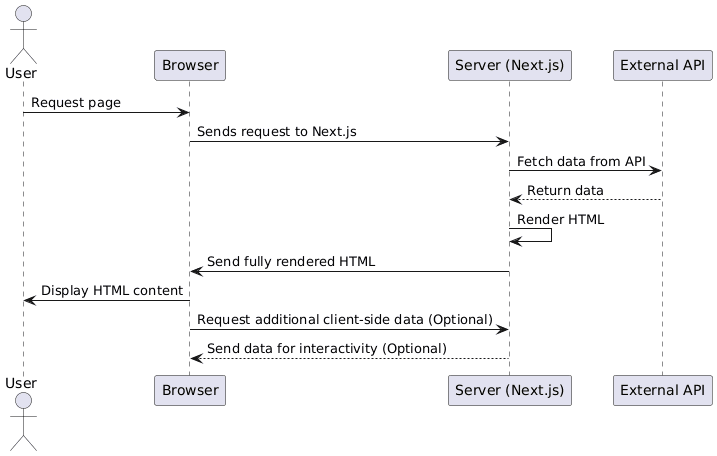
Server-Side Rendering (SSR) is a crucial technique for building fast, scalable, and SEO-friendly web applications. While client-side rendering has been the traditional approach for React applications, the demand for better performance and improved SEO has pushed developers to embrace SSR. In this article, we’ll dive into the world of SSR using Next.js, one of the most popular frameworks that enable SSR out of the box. We will walk through a beginner-friendly guide, complete with code snippets and best practices.
Introduction: Why Server-Side Rendering?
In client-side rendering (CSR), JavaScript runs on the user’s browser to generate the HTML for the page. This often leads to slower page load times, especially on large applications, as users must wait for JavaScript to be downloaded and executed. Moreover, CSR can negatively impact SEO, as search engine crawlers may not fully execute JavaScript.
Server-Side Rendering (SSR), on the other hand, generates HTML on the server before sending it to the client. This allows the browser to display the content faster, leading to a better user experience. Additionally, SSR makes web pages more search-engine friendly since crawlers receive fully-rendered HTML.
Why Use Next.js for SSR in React?
While React is great for building interactive user interfaces, implementing SSR manually can be challenging. This is where Next.js comes into play. Next.js, a React framework, simplifies SSR by providing built-in support for it. Besides SSR, Next.js offers features like static site generation, dynamic routing, and file-based routing, making it the go-to framework for modern React developers.
Next.js automatically decides when to render a page on the server and when to cache it for static delivery, combining the best of both SSR and static site generation (SSG).
Client-Side Rendering (CSR) vs. SSR: When to Use SSR?
SSR shines in scenarios where:
SEO is a priority (e.g., blogs, news websites).
Performance matters, especially for initial page loads.
You want to enhance user experience with a fast-loading site that feels interactive right away.
For static pages or content that doesn’t change frequently, static site generation (SSG) might be a better option, while SSR is great for dynamic content.
Getting Started with Next.js
Let’s get started by creating a simple Next.js application:
Install Next.js by running the following command:
npx create-next-app@latest my-ssr-app cd my-ssr-app npm run dev
This will set up a Next.js project and run a development server at http://localhost:3000.
File-based Routing: In Next.js, pages are automatically created based on files inside the pages/ directory. Create a new file called index.js:
// pages/index.js export default function Home() { return ( <div> <h1>Welcome to My SSR App</h1> </div> ); }
When you visit http://localhost:3000, you will see the above page, and it's rendered server-side.
Implementing SSR in a Basic React Component
To use SSR, we need to fetch data on the server before the page is rendered. Next.js provides the getServerSideProps() function for this purpose. Let's implement it:
// pages/data.js
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return { props: { data } };
}
export default function DataPage({ data }) {
return (
<div>
<h1>Server-Side Rendered Data</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
In this example:
getServerSideProps() fetches data on the server.
The fetched data is then passed to the DataPage component as props, allowing it to be rendered before being sent to the client.
This results in a faster page load, especially for users with slow connections, as they receive the fully-rendered HTML from the server.
Performance Benefits of SSR
By rendering the initial page on the server, SSR reduces Time to First Byte (TTFB) and improves the First Contentful Paint (FCP) metric. This means users see content sooner, improving perceived performance.
Additionally, SSR helps with situations where JavaScript execution might be blocked or delayed, providing a more robust user experience. Combined with client-side hydration, SSR offers the best of both worlds—fast initial loads and the ability to handle complex interactivity on the client side.
SEO Advantages of SSR
With SSR, search engines receive fully-rendered HTML, making it easier for crawlers to index the content. Let’s enhance our previous example by dynamically generating SEO metadata:
import Head from 'next/head';
export default function SEOPage({ data }) {
return (
<>
<Head>
<title>{data.title} - My App</title>
<meta name="description" content={data.description} />
</Head>
<div>{data.content}</div>
</>
);
}
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/page');
const data = await res.json();
return { props: { data } };
}
In this example:
The <Head> component in Next.js allows us to manage metadata dynamically.
The SEO metadata is sent directly to the browser and search engines, improving the page's visibility in search results.
Best Practices for SSR in Production
To ensure optimal performance in production, follow these best practices:
Caching: Cache API responses or render results where possible to avoid redundant server processing.
Content Delivery Network (CDN): Use CDNs to serve static assets (e.g., images, CSS) to reduce latency and improve load times.
Optimize Server Response: Ensure your server responds quickly by optimizing data-fetching functions and server logic.
Lazy Loading: Use lazy loading for components or data that aren’t needed immediately, improving both performance and user experience.
Conclusion
Server-Side Rendering is a powerful technique for building performant and SEO-optimized web applications, especially when paired with React and Next.js. By fetching data on the server and delivering fully-rendered HTML, SSR can significantly improve the performance of dynamic web applications.
Next.js simplifies SSR implementation, allowing developers to focus on building great user experiences with minimal setup. Whether you're building a blog, e-commerce site, or complex web application, SSR should be part of your toolkit to ensure your website performs at its best.
By following this guide, you now have a solid understanding of how to implement SSR in a React application using Next.js, with real-world examples and best practices to get you started.
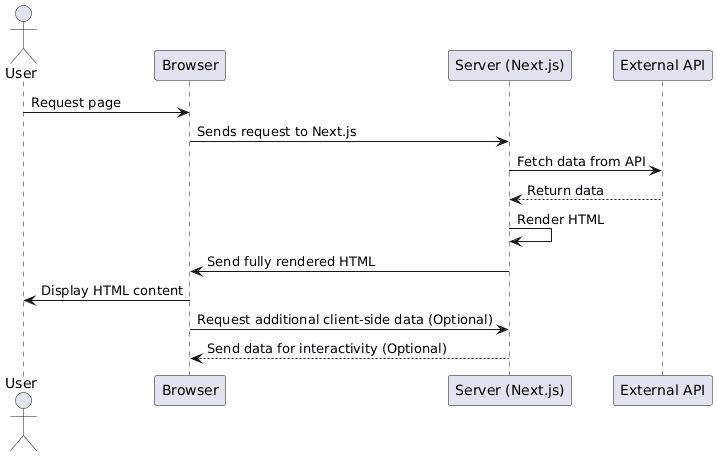
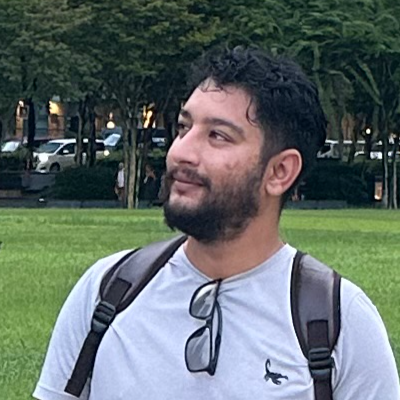
Kiran Chaulagain
kkchaulagain@gmail.com
I am a Full Stack Software Engineer and DevOps expert with over 6 years of experience. Specializing in creating innovative, scalable solutions using technologies like PHP, Node.js, Vue, React, Docker, and Kubernetes, I have a strong foundation in both development and infrastructure with a BSc in Computer Science and Information Technology (CSIT) from Tribhuvan University. I’m passionate about staying ahead of industry trends and delivering projects on time and within budget, all while bridging the gap between development and production. Currently, I’m exploring new opportunities to bring my skills and expertise to exciting new challenges.