Understanding the Difference Between Controlled and Uncontrolled Components in React
Listen to article Podcast:
This article compares and contrasts the two primary approaches to handling form data in React: controlled and uncontrolled components. Controlled components use React's state to manage form data, resulting in predictable behavior, real-time validation, and easier debugging. Uncontrolled components rely on the DOM to store input values, offering a simpler implementation for basic forms. The article explains the characteristics of each approach, provides code examples, and discusses the advantages and disadvantages of each method. The article concludes with guidelines on when to use each type of component based on the complexity of the form and desired features.
React, as a popular front-end library, offers different approaches for managing form inputs. The two primary ways to handle form data in React are controlled components and uncontrolled components. Knowing the difference between these two methods is crucial for managing form inputs effectively and ensuring your application's behavior is predictable and easy to debug.
In this article, we'll dive deep into the concepts of controlled and uncontrolled components, explain how they work, and give practical examples to illustrate their differences.
What Are Controlled Components in React?
In React, controlled components are form elements (like input fields, checkboxes, text areas, etc.) that are controlled by React’s state. This means that the value of these form elements is determined by the component’s state, making React the "single source of truth" for the form data.
Key Characteristics of Controlled Components:
State Management: Form input values are stored in the component’s state. When a user types or interacts with an input, the onChange event updates the state, and the component re-renders with the new state value.
Predictability: Since the UI is always in sync with the state, it’s easy to track and control the input values. This ensures the component behaves consistently.
Real-Time Input Handling: You can easily validate user input, implement conditional rendering, or trigger actions based on the input as you have real-time control over the input values.
Example of a Controlled Component:
Here’s an example of how to create a controlled text input in React:
import React, { useState } from 'react';
function ControlledComponent() {
// Declare a state variable to hold the input value
const [inputValue, setInputValue] = useState('');
// Function to handle input changes
const handleChange = (event) => {
setInputValue(event.target.value); // Update state when the user types
};
return (
<div>
<h2>Controlled Input</h2>
<input
type="text"
value={inputValue} // The input value comes from state
onChange={handleChange} // Update the state on every keystroke
/>
<p>Current value: {inputValue}</p>
</div>
);
}
In this example:
The input field’s value is controlled by the state (inputValue).
When the user types in the input field, the onChange handler updates the state using setInputValue() .
As the state changes, the input field re-renders with the updated value.
Advantages of Controlled Components:
Full Control Over Input: You can easily validate user input, restrict certain characters, or perform side effects (e.g., enabling/disabling a submit button).
React as the Single Source of Truth: All the data lives in the component’s state, making debugging and maintaining the code easier.
Drawbacks:
Increased Boilerplate: For every input field, you need to maintain state and write event handlers, which can lead to more code, especially in complex forms.
Re-renders: Controlled components re-render on every input change, which can be less efficient if not optimized correctly in large forms.
What Are Uncontrolled Components in React?
On the other hand, uncontrolled components allow form elements to maintain their own state. Instead of relying on React’s state, uncontrolled components use the DOM to store input values. In this case, React only accesses the values when needed, typically at the time of form submission.
Key Characteristics of Uncontrolled Components:
DOM-Centric: The value of the form input is stored directly in the DOM. React does not manage or keep track of the value.
Refs for Access: To retrieve or manipulate the value of an uncontrolled component, you use Refs (a way to reference a DOM element directly in React).
Simpler Implementation: Uncontrolled components can be easier to implement for simple use cases where real-time input tracking is not necessary (e.g., basic forms or data collection where you only need the value upon submission).
Example of an Uncontrolled Component:
Here’s an example of an uncontrolled text input using useRef to access the DOM directly:
import React, { useRef } from 'react';
function UncontrolledComponent() {
// Create a ref to store the input DOM element
const inputRef = useRef(null);
// Function to handle form submission
const handleSubmit = (event) => {
event.preventDefault();
// Access the input's value directly from the DOM using the ref
console.log("Submitted value:", inputRef.current.value);
};
return (
<div>
<h2>Uncontrolled Input</h2>
<form onSubmit={handleSubmit}>
<input
type="text"
ref={inputRef} // Use the ref to access the input value later
/>
<button type="submit">Submit</button>
</form>
</div>
);
}
In this example:
The input’s value is not managed by React’s state. Instead, the value is directly stored in the DOM.
The useRef hook creates a reference to the input DOM element.
When the form is submitted, the value of the input is accessed via inputRef.current.value.
Advantages of Uncontrolled Components:
Less Boilerplate: No need to maintain state or write event handlers for every input.
Simpler for Basic Forms: Suitable for simpler forms where real-time validation or interaction is not required.
Drawbacks:
Less Predictable: Since React doesn’t manage the input value, the component’s behavior may be less predictable, especially in more complex forms.
More Difficult to Implement Features: Implementing features like validation, conditional rendering, or real-time input changes becomes more cumbersome.
Key Differences Between Controlled and Uncontrolled Components
Feature | Controlled Components | Uncontrolled Components |
State Management | Managed by React state | Managed by the DOM |
Value Access | Accessed via state | Accessed via refs |
Predictability | Highly predictable | Less predictable, React has no control |
Form Validation | Easier to implement | More challenging |
Use Case | Preferred for complex forms | Suitable for simple forms |
Boilerplate Code | More code needed (state, handlers) | Less code (no state required) |
Reactivity | Changes are tracked and reflected in real-time | No real-time tracking of changes |
When Should You Use Controlled or Uncontrolled Components?
Use Controlled Components When:
You need real-time validation (e.g., showing an error message as the user types).
You require conditional rendering or complex form behavior (e.g., disabling a button until all fields are filled correctly).
You want full control over the form’s data and need to update other parts of the UI based on the form inputs.
You are dealing with complex forms where multiple fields need to interact or depend on each other.
Use Uncontrolled Components When:
The form is simple and you only need the value once, typically at submission time.
You want to minimize the amount of code for basic forms (e.g., newsletter sign-ups or login forms).
You are integrating with non-React code or third-party libraries that require uncontrolled inputs.
For instance, if you're building a basic contact form where you only need to collect the input upon form submission, an uncontrolled component can be a simpler and more efficient choice. However, if you're building a more complex form with validation and interdependent fields, a controlled component would be the better option for maintaining predictability and ease of control.
Conclusion
In React, choosing between controlled and uncontrolled components depends on your specific use case. Controlled components offer better control, predictability, and real-time handling of form data, making them ideal for complex forms. Uncontrolled components, while less powerful, are simpler to implement and may be appropriate for basic forms where you don’t need continuous input tracking.
By understanding these two approaches and knowing when to use each, you can write more efficient and maintainable React applications, ensuring your forms behave as expected. Here are the references for the article:
These links offer further insights into controlled and uncontrolled components, providing additional resources for those who want to explore the topic more deeply.
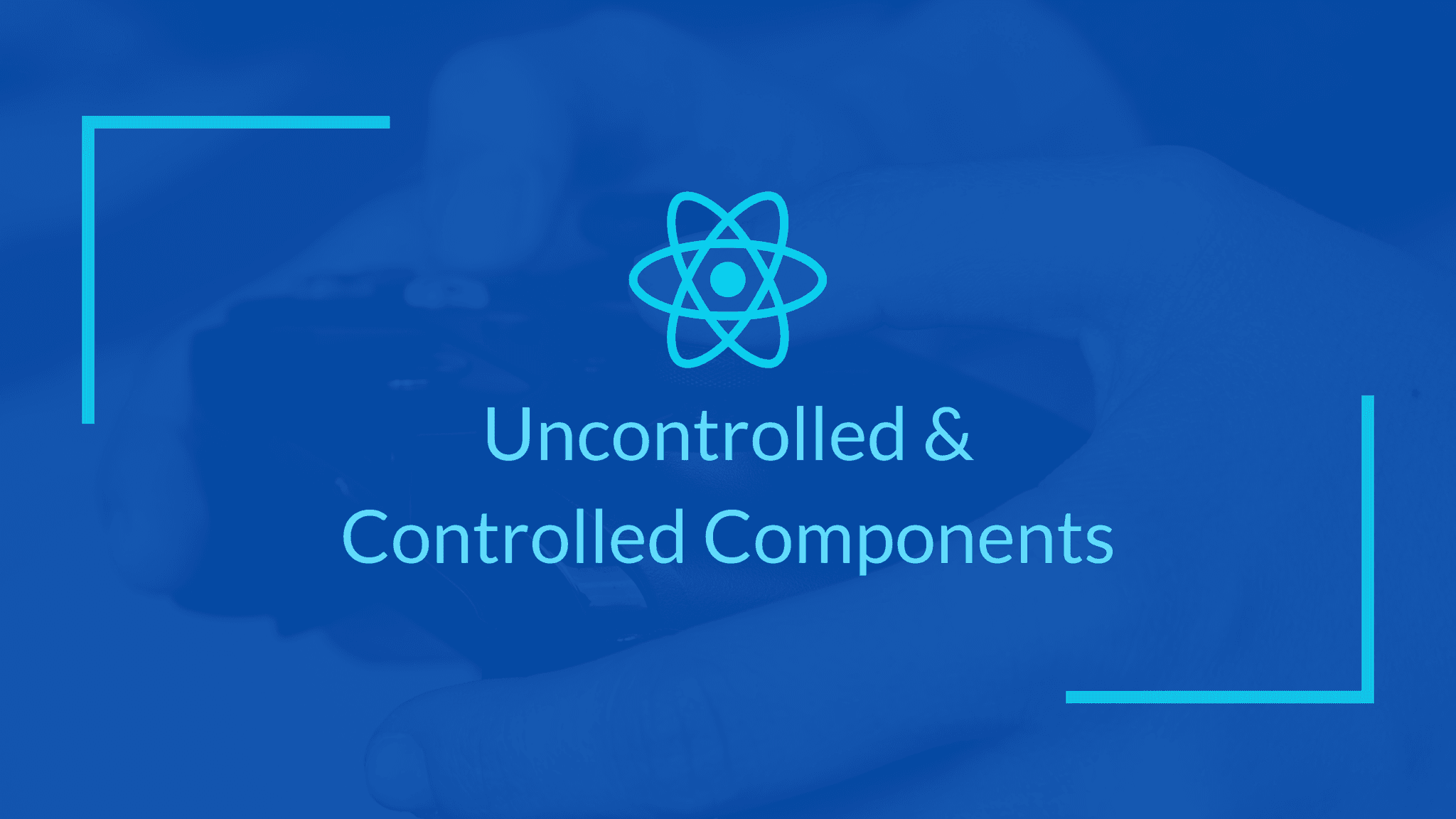
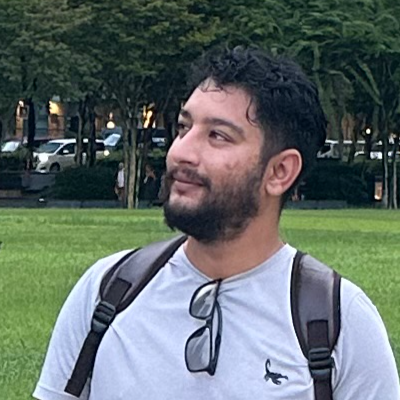
Kiran Chaulagain
kkchaulagain@gmail.com
I am a Full Stack Software Engineer and DevOps expert with over 6 years of experience. Specializing in creating innovative, scalable solutions using technologies like PHP, Node.js, Vue, React, Docker, and Kubernetes, I have a strong foundation in both development and infrastructure with a BSc in Computer Science and Information Technology (CSIT) from Tribhuvan University. I’m passionate about staying ahead of industry trends and delivering projects on time and within budget, all while bridging the gap between development and production. Currently, I’m exploring new opportunities to bring my skills and expertise to exciting new challenges.