Writing Effective Unit Tests in Python with Pytest: A Practical Guide to Testing FastAPI Endpoints
Writing Effective Unit Tests in Python with Pytest: A Practical Guide to Testing FastAPI Endpoints
Welcome, fellow Python enthusiasts! If you're building applications, especially with a modern framework like FastAPI, you've likely heard the term "testing." Maybe it sounds a bit intimidating, or maybe you just aren't sure where to start.
Well, you're in the right place! In this guide, we're going to demystify unit testing and show you how to write effective tests for your FastAPI application's endpoints using the fantastic Pytest framework. We'll cover the essentials, from setting up Pytest to simulating API requests, handling async code, mocking dependencies, and even using FastAPI's powerful dependency override feature.
Let's dive in!
Introduction: Why Test? Why Pytest?
First off, why bother with testing? Isn't writing the code itself enough?
Think of testing as building safety nets for your code. Here's why it's crucial:
Catch Bugs Early:
The most obvious benefit. Tests can find issues before your users do. This saves you time, effort, and potential headaches down the line.
Enable Refactoring:
Want to change how a part of your code works? With tests, you can confidently refactor (restructure code without changing its external behavior) knowing that if you break something, your tests will tell you.
Act as Documentation:
Well-written tests show how different parts of your code are intended to be used. They serve as living examples.
Now, why Pytest? Python has built-in testing capabilities (
unittest
Simplicity: Writing tests in Pytest often feels like writing regular Python functions.
Powerful Fixtures: A flexible and powerful way to set up resources (like database connections, test clients, or mock objects) for your tests.
Rich Ecosystem: Lots of helpful plugins are available to extend its functionality.
Readability: Tests written with Pytest are generally easy to read and understand.
Getting Started with Pytest
Let's get Pytest set up in your project.
Installation:
We'll need Pytest itself, plus a few handy plugins for testing asynchronous code and mocking, and of course, FastAPI and a library to simulate requests (
httpx
TestClient
Open your terminal in your project directory and run:
pip install pytest pytest-asyncio pytest-mock fastapi httpx
How Pytest Discovers Tests:
By default, Pytest is smart. It automatically finds tests in:
Files named test_*.py or *_test.py.
Functions or methods within those files named test_*
.
This simple convention means you usually don't need any extra configuration for Pytest to find your tests.
Basic Test Function Structure:
A basic Pytest test function is just a Python function whose name starts with test_. Inside, you use the standard Python assert statement to check if something is true. If an
assert
# Inside a file named test_example.py
def test_addition():
"""Tests that 1 + 1 equals 2."""
assert 1 + 1 == 2
def test_string_contains():
"""Tests if a substring is present."""
greeting = "Hello, World!"
assert "Hello" in greeting
def test_list_length():
"""Tests the number of items in a list."""
my_list = [1, 2, 3]
assert len(my_list) == 3
You can run these tests by simply navigating to your project directory in the terminal and typing pytest.
Core Pytest Concepts
Let's look at two fundamental building blocks in Pytest: Assertions and Fixtures.
Assertions:
Pytest uses the standard Python
assert
Pytest provides helpful output when an assertion fails, showing you the values of the variables involved.
# Example of a failing assertion
def test_subtraction():
"""This test will fail!"""
assert 5 - 2 == 4 # Expecting 3, asserting 4
When you run this test, Pytest will show you something like assert 3 == 4, making it clear what went wrong.
You can assert equality (==), inequality (!=), comparisons (<, >, <=, >=), membership (in, not in), identity (is, is not), and even that a specific exception is raised using pytest.raises.
Fixtures (@pytest.fixture
Fixtures are one of Pytest's most powerful features. Their purpose is to provide a reliable baseline, resources, or setup for your tests. Think of them as setup functions that prepare the environment or data needed by one or more tests.
You define a fixture using the @pytest.fixture decorator. Tests that need the resource provided by a fixture simply include the fixture function's name as an argument. Pytest automatically detects this and runs the fixture before executing the test.
import pytest
@pytest.fixture
def sample_data():
"""A fixture providing some sample data."""
data = {"name": "Test User", "id": 123}
print("\n--- Setting up sample_data ---") # Runs before test
yield data # The data is available to the test
print("\n--- Tearing down sample_data ---") # Runs after test (if yield is used)
def test_process_data(sample_data):
"""This test uses the sample_data fixture."""
# The 'sample_data' argument receives the value yielded by the fixture
assert sample_data["name"] == "Test User"
assert sample_data["id"] == 123
The
yield
yield
Fixtures have different scopes:
function(default): The fixture runs once for each test function that uses it.
class: The fixture runs once per test class.
module: The fixture runs once per test module (.py file).
session: The fixture runs once per Pytest session (the entire test run).
The
client
Testing Web APIs with TestClient (FastAPI Specific)
When testing a web API, you want to simulate sending HTTP requests (GET, POST, etc.) to your endpoints and checking the responses (status code, headers, body). FastAPI provides a built-in tool for this:
TestClient
The TestClient from fastapi.testclient allows you to make synchronous requests to your FastAPI application without actually running a server. It's incredibly fast and convenient for testing.
You typically create an instance of TestClient pointed at your FastAPI
app
# In your test file (e.g., test_api.py)
from fastapi.testclient import TestClient
from your_app import app # Assuming your FastAPI app instance is called 'app' in your_app.py
import pytest
# A fixture to provide the test client
@pytest.fixture
def client():
"""Provides a TestClient instance for the FastAPI app."""
with TestClient(app) as c:
yield c # The TestClient instance
Now, any test function that includes client as an argument will receive this TestClient instance. You can use it to make requests:
def test_read_main(client):
"""Tests the root endpoint."""
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"message": "Hello World"}
def test_post_data(client):
"""Tests a POST endpoint."""
test_payload = {"item_id": "baz", "quantity": 5}
response = client.post("/items/", json=test_payload) # Use json= for JSON bodies
assert response.status_code == 200
# Check the response body
response_data = response.json()
assert response_data["item_id"] == "baz"
assert response_data["quantity"] == 5
You can access the response details using:
response.status_code: The HTTP status code (e.g., 200, 404, 422).
response.json(): Parses the response body as JSON.
response.text: The raw response body as a string.
response.headers: A dictionary-like object of response headers.
The calls like
client.post("
", json=...)
TestClient
Handling Asynchronous Code (async
/await
)
async
await
FastAPI is built on asynchronous Python (
asyncio
async def
await
The
pytest-asyncio
Add
@pytest.mark.asyncio
decorator to your
async def
test functions.
Use
await
when calling other
async def
functions or methods inside your test.
import pytest
from fastapi.testclient import TestClient
from your_async_app import app # Assuming an app with async endpoints
# Need pytest-asyncio installed
pytestmark = pytest.mark.asyncio # Can apply to all tests in the file
@pytest.fixture
def client():
"""Provides a TestClient instance for the FastAPI app."""
with TestClient(app) as c:
yield c
async def async_helper_function():
"""A dummy async function."""
await asyncio.sleep(0.01) # Simulate some async work
return "done"
async def test_async_endpoint(client):
"""Tests an async endpoint."""
response = client.get("/async-path") # TestClient handles calling async endpoints
assert response.status_code == 200
assert response.json() == {"message": "Async response"}
async def test_calling_async_helper():
"""Tests calling an async helper."""
result = await async_helper_function() # Need await here
assert result == "done"
# async def test_register_births_validation_error(...): # Your example test would be async
# async def test_register_births_api(...): # Your example test would be async
The
TestClient
client.get()
client.post()
async def
await
AsyncMock
@pytest.mark.asyncio
Mocking Dependencies
In unit testing, we aim to test a small "unit" of code in isolation. Often, this unit depends on other things: a database connection, an external API call, reading a file, or even just another Python function/class.
Mocking is the technique of replacing these dependencies with controlled "mock" objects during the test. This allows you to:
Isolate the Unit:
Test only the code you intend to test, without worrying if the database is down or the external API is slow.
Control Behavior:
Make the dependency return specific values or raise specific errors to test different scenarios (e.g., what happens if the database returns nothing, or the external API returns an error?).
Avoid Side Effects:
Prevent tests from actually writing to a database, sending emails, etc.
Python's standard library includes the
unittest.mock
pytest-mock
mocker
The most common operation is
mocker.patch()
Mock
AsyncMock
# Assuming you have a function somewhere:
# def get_external_data(item_id):
# # Makes an external call...
# pass
def process_item(item_id):
# This function calls get_external_data
data = get_external_data(item_id)
return data * 2 # Simple processing
def test_process_item_with_mock(mocker):
"""Tests process_item by mocking get_external_data."""
# Patch the get_external_data function where it's *used* or *imported*
# Use the full path: module.submodule.function_name
mock_external_data = mocker.patch("your_module.get_external_data")
# Configure the mock's return value
mock_external_data.return_value = 10
# Call the function under test
result = process_item("test_item")
# Assert the function did its job with the mocked data
assert result == 20 # (10 * 2)
# Optionally, assert that the mocked function was called
mock_external_data.assert_called_once_with("test_item")
In your example, the
mock_get_animal_controller
mocker.patch
return_value
AsyncMock
You'd need
AsyncMock
async def
return_value
side_effect
AsyncMock
FastAPI Specific: Dependency Overrides
FastAPI has a powerful feature specifically designed to make testing dependencies easier:
app.dependency_overrides
You know how FastAPI uses dependency injection to provide resources (like database sessions, current user objects, or configuration settings) to your path operations?
dependency_overrides
This is incredibly useful for:
Mocking Authentication:
Replace the function that checks for a valid user token to simulate logged-in or logged-out states without needing real credentials.
Injecting Mock Services:
Replace a dependency that provides a database connection or an external service client with a mock object.
The pattern is:
Store the original dependency provider function.
Set
app.dependency_overrides[OriginalDependencyProvider]
to your
MockDependencyProvider
.
Make the test request using the
TestClient
.
Crucially:
Clean up the overrides in a
finally
block to ensure the original dependencies are restored for subsequent tests. This guarantees test isolation.
# In your test file
from fastapi.testclient import TestClient
from your_app import app # Your FastAPI app
from your_app.dependencies import verify_firebase_token # The original dependency
# A simple mock dependency function
def mock_verify_firebase_token():
"""A mock that pretends the user is authenticated."""
# Return whatever the original dependency would return on success
return {"uid": "mock_user_id", "email": "mock@example.com"}
# Or maybe a mock that raises an exception for unauthenticated
def mock_unauthorized():
from fastapi import HTTPException, status
raise HTTPException(status_code=status.HTTP_403_FORBIDDEN, detail="Not authorized")
def test_some_endpoint_authenticated(client):
"""Tests an endpoint that requires authentication."""
original_dependency = app.dependency_overrides.get(verify_firebase_token)
try:
# Override the dependency for this test
app.dependency_overrides[verify_firebase_token] = mock_verify_firebase_token
# Make the request - it will now use the mock dependency
response = client.get("/secure-endpoint")
# Assert the expected outcome for an authenticated user
assert response.status_code == 200
# ... check response body ...
finally:
# ALWAYS clean up dependency overrides!
if original_dependency:
app.dependency_overrides[verify_firebase_token] = original_dependency
else:
del app.dependency_overrides[verify_firebase_token] # Remove if it wasn't there initially
# A simpler way is often just to clear the whole dict if you know you only set overrides in tests:
# app.dependency_overrides.clear()
def test_some_endpoint_unauthorized(client):
"""Tests an endpoint with a mock that causes unauthorized access."""
original_dependency = app.dependency_overrides.get(verify_firebase_token)
try:
app.dependency_overrides[verify_firebase_token] = mock_unauthorized
response = client.get("/secure-endpoint")
assert response.status_code == 403 # Check for Forbidden
finally:
if original_dependency:
app.dependency_overrides[verify_firebase_token] = original_dependency
else:
del app.dependency_overrides[verify_firebase_token]
# Or app.dependency_overrides.clear()
In your example, using
app.dependency_overrides
verify_firebase_token
HTTPException
Conclusion
Phew! We've covered a lot of ground. You've learned:
Why testing is essential and how Pytest helps.
The basics of setting up Pytest and writing simple tests.
Using
assert
for checks and fixtures for test setup.
Simulating API requests with FastAPI's
TestClient
.
Handling asynchronous tests with
pytest-asyncio
.
Isolating your code using mocking with
pytest-mock
.
Leveraging FastAPI's
dependency_overrides
for easy testing of dependencies like authentication.
Testing might seem like extra work initially, but integrating it into your development process from the start will save you significant time and stress in the long run. It gives you confidence to build, refactor, and deploy your FastAPI applications.
This is just the beginning! You can explore more advanced Pytest features like parameterization (running the same test with different inputs), testing database interactions more thoroughly, and measuring your test coverage to see how much of your code is being tested.
Now, go forth and write some tests! Your future self (and your users) will thank you.
Happy Testing!
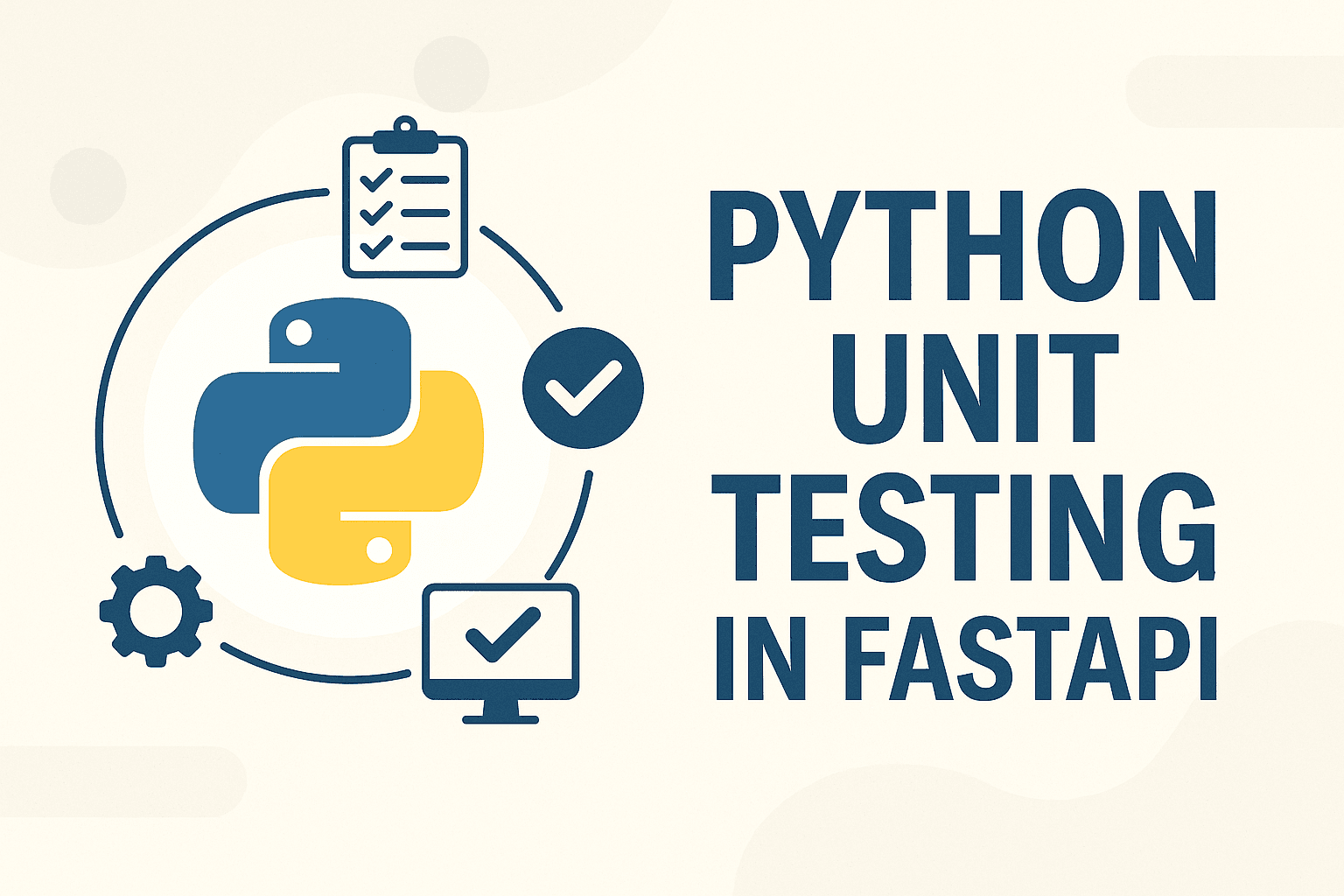
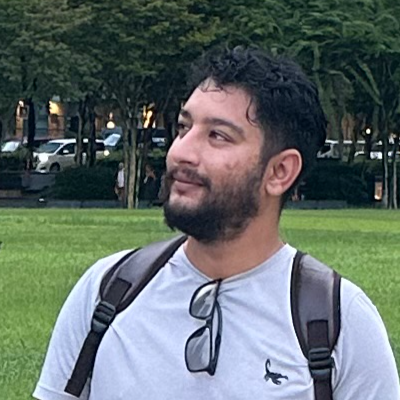
Kiran Chaulagain
kkchaulagain@gmail.com
I am a Full Stack Software Engineer and DevOps expert with over 6 years of experience. Specializing in creating innovative, scalable solutions using technologies like PHP, Node.js, Vue, React, Docker, and Kubernetes, I have a strong foundation in both development and infrastructure with a BSc in Computer Science and Information Technology (CSIT) from Tribhuvan University. I’m passionate about staying ahead of industry trends and delivering projects on time and within budget, all while bridging the gap between development and production. Currently, I’m exploring new opportunities to bring my skills and expertise to exciting new challenges.